Hello,
I have the issue that I cannot read an item with the InventoryConnector as I am getting always the following exception:
com.clover.sdk.v1.ForbiddenException: status code: 403 Permission denied
In the beginning I had a German account (it was also not working) which I have now changed to an US account as there are more options available.
Here is what I have done:
Modules which my app requires (I have checked everything just to be be sure):
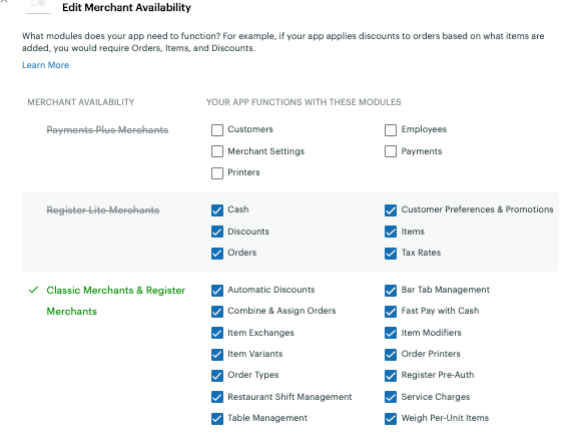
A simple app which is mainly the same as the example code:
import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import androidx.core.content.ContextCompat; import android.Manifest; import android.accounts.Account; import android.app.Activity; import android.content.pm.PackageManager; import android.os.AsyncTask; import android.os.Bundle; import android.os.RemoteException; import android.view.View; import android.widget.TextView; import com.clover.sdk.util.CloverAccount; import com.clover.sdk.v1.BindingException; import com.clover.sdk.v1.ClientException; import com.clover.sdk.v1.ServiceException; import com.clover.sdk.v3.inventory.InventoryConnector; import com.clover.sdk.v3.inventory.Item; public class MainActivity extends AppCompatActivity { private Account mAccount; private InventoryConnector mInventoryConnector; private TextView mTextView; private static final int MY_PERMISSIONS_REQUEST_READ_CONTACTS = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mTextView = (TextView) findViewById(R.id.textView); } private class InventoryAsyncTask extends AsyncTask<Void, Void, Item> { @Override protected final Item doInBackground(Void... params) { try { //Get inventory item //return mInventoryConnector.getItems().get(0); Item item; item = mInventoryConnector.getItems().get(0); return item; } catch (RemoteException | ClientException | ServiceException | BindingException e) { //mTextView.setText("Inventory permissions missing"); e.printStackTrace(); } return null; } @Override protected final void onPostExecute(Item item) { if (item != null) { mTextView.setText(item.getName()); } else { mTextView.setText("an error happened when getting the item"); } } } public void btnStart_onClick(View view) { mTextView.setText("starting to get an item ..."); // Connect InventoryConnector connect(); // Get Item new InventoryAsyncTask().execute(); } @Override protected void onResume() { super.onResume(); Activity thisActivity = this; // Here, thisActivity is the current activity if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.GET_ACCOUNTS) != PackageManager.PERMISSION_GRANTED) { // No explanation needed; request the permission ActivityCompat.requestPermissions(thisActivity, new String[]{Manifest.permission.GET_ACCOUNTS}, MY_PERMISSIONS_REQUEST_READ_CONTACTS); } if (mAccount == null) { mAccount = CloverAccount.getAccount(this.getApplicationContext()); if (mAccount == null) { mTextView.setText("Could not get account :-("); return; } mTextView.setText("Got account"); } } @Override protected void onDestroy() { super.onDestroy(); disconnect(); } private void connect() { disconnect(); if (mAccount != null) { mInventoryConnector = new InventoryConnector(this, mAccount, null); mInventoryConnector.connect(); } } private void disconnect() { if (mInventoryConnector != null) { mInventoryConnector.disconnect(); mInventoryConnector = null; } } }
The app is signed and uploaded to the sandbox environment. I installed the app via the App Updater.
Additionally I have synced the user account (several times).
According to the Inventory app there is one item.
I have also tried the REST API and it returns the correct information:
{ "elements":[ 0:{ "id":"GMPNG7WJJ2474" "hidden":false "name":"Wasser" "alternateName":"" "code":"" "sku":"" "price":100 "priceType":"FIXED" "defaultTaxRates":true "unitName":"" "cost":50 "isRevenue":true "modifiedTime":1586200568000 } ]
Could you please help me to understand where my mistake is?
Thank you
Philipp